Why you should use Python's dataclasses instead of regular classes
If you are looking for a way to make your code more concise and efficient, dataclasses are a great choice.
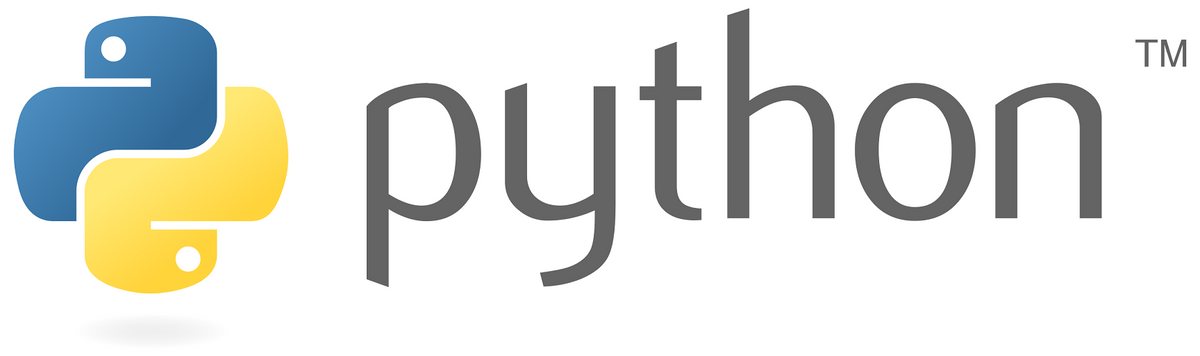
Python dataclasses are a relatively new feature (added in Python 3.7) that make it easier to create classes that primarily hold data. These classes offer several advantages over regular classes in Python, making them a powerful tool for organizing and manipulating data in your programs.
The development of dataclasses began in 2017 with PEP 557 and was accepted in Python 3.7. It was aimed to make working with simple classes that only store data more convenient. The main motivation behind the inclusion of dataclasses was the explosion of usage of Python in data science, where a large amount of data is stored in classes and the amount of boilerplate code needed to define these classes was often cumbersome. The first draft of the PEP was authored by Eric V. Smith, and was later reviewed, refined and finalized by Guido van Rossum and other core developers of Python.
In the following, we discuss the benefits of using dataclasses and why they are a great choice for your next data science project.
Simplified class definition
Dataclasses provide a concise syntax for defining classes that represent data. They automatically generate special methods such as __init__
, __repr__
, and __eq__
, which would otherwise have to be implemented manually in a regular class.
For example, look at this piece of code:
# Regular class
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __repr__(self):
return f"Person(name='{self.name}', age={self.age})"
def __eq__(self, other):
if isinstance(other, Person):
return self.name == other.name and self.age == other.age
return False
p = Person("John", 30)
print(p) # Person(name='John', age=30)
# Dataclass
from dataclasses import dataclass
@dataclass
class Person:
name: str
age: int
p = Person("John", 30)
print(p) # Person(name='John', age=30)
As you can see, with dataclasses the __init__
, __repr__
, and __eq__
methods are automatically generated, reducing the amount of code required and making it more readable. Additionally, dataclasses have some other features, like default values and type hints, which makes them more powerful and useful.
Improved readability
One of the main benefits of dataclasses is that they can make your code more readable, especially if you have a lot of data that needs to be stored and accessed.
For example, consider the following code:
from dataclasses import dataclass
@dataclass
class Employee:
name: str
age: int
salary: float
department: str
position: str
employee1 = Employee("John Smith", 35, 50000.0, "IT", "Developer")
employee2 = Employee("Jane Doe", 28, 45000.0, "Marketing", "Manager")
print(employee1) # prints "Employee(name='John Smith', age=35, salary=50000.0, department='IT', position='Developer')"
print(employee2) # prints "Employee(name='Jane Doe', age=28, salary=45000.0, department='Marketing', position='Manager')"
In this example, we have a class called Employee
that stores information about an employee, including their name, age, salary, department, and position. By using the @dataclass
decorator, we can automatically create an __init__
method that sets up the class and also a __repr__
method that provides a string representation of an instance of the class.
It's clear that the class structure is simple and easy to understand, you can easily understand that the class is holding data about Employee
and the data fields are clearly defined with their types. Also, when creating the instance of the class, it's clear what values are passed for each attribute. This makes the code more readable, which is especially useful if you have a lot of data that needs to be stored and accessed.
Furthermore, the __repr__
method created by the decorator makes it easy to print the instances of the class, it gives a clear output of the data stored in the object. This makes debugging and working with the objects in the interactive interpreter easy.
Improved maintainability
Dataclasses can also make your code more maintainable, as they allow you to define the data for your objects in a single place, rather than scattering it throughout your code. This can be especially helpful if you need to add, remove, or change the data for your objects in the future.
For example, consider the following code:
# Using regular class
class Employee:
def __init__(self, name, age, salary, department, position):
self.name = name
self.age = age
self.salary = salary
self.department = department
self.position = position
def increase_salary(self, amount):
self.salary += amount
employee1 = Employee("John Smith", 35, 50000.0, "IT", "Developer")
employee1.increase_salary(1000)
# Using dataclass
from dataclasses import dataclass
@dataclass
class Employee:
name: str
age: int
salary: float
department: str
position: str
employee1 = Employee("John Smith", 35, 50000.0, "IT", "Developer")
In the first example, we have a regular class called Employee
that has an __init__
method that sets up the class, and also a method increase_salary
that increases the salary of an employee.
If you need to add, remove or change the data for the Employee
class, you will have to edit the __init__
method and also all the other methods that use that attribute. This can be time-consuming and error-prone, especially if the class is used in multiple places in your codebase.
In the second example, we used a dataclass Employee
that has the same attributes and the type hints for them. The use of the @dataclass
decorator automatically created an __init__
method that sets up the class and also a __repr__
method that provides a string representation of an instance of the class.
If you need to add, remove or change the data for the Employee
class, you can simply add it to the class definition, and it will be automatically added to the __init__
method, without you having to change any other part of the code. This makes the code more maintainable, as the data for your objects is defined in a single place, rather than scattered throughout your code.
Improved type safety
Dataclasses can also improve the type safety of your code, as they allow you to define the data types for your objects in a single place, rather than scattering them throughout your code. This can be especially helpful in cases where you need to ensure that your objects have the correct data types.
For example, consider the following code:
# Using regular class
class Employee:
def __init__(self, name, age, salary, department, position):
self.name = name
self.age = age
self.salary = salary
self.department = department
self.position = position
def increase_salary(self, amount):
if type(amount) is not float:
raise TypeError("amount should be of type float")
self.salary += amount
employee1 = Employee("John Smith", 35, 50000.0, "IT", "Developer")
employee1.increase_salary(1000)
# Using dataclass
from dataclasses import dataclass
@dataclass
class Employee:
name: str
age: int
salary: float
department: str
position: str
employee1 = Employee("John Smith", 35, 50000.0, "IT", "Developer")
# If someone passes an int instead of a float, it will raise an error
# "TypeError: Argument 'salary' has incorrect type (expected float, got int)"
In the first example, we have a regular class called Employee
that has an init method that sets up the class, and also a method increase_salary that increases the salary of an employee. In the method, we check if the type of amount passed is a float. If it is not, we raise a TypeError
. This is an explicit way of ensuring the type safety of the data, but it can be error-prone and repetitive if the same check is needed in multiple places in the code.
In the second example, we used a dataclass Employee
that has the same attributes and the type hints for them. The use of the @dataclass
decorator automatically created an __init__
method that sets up the class and also a __repr__
method that provides a string representation of an instance of the class.
By providing type hints in the class definition, the dataclasses module ensures that the arguments passed to the initializer have the correct types. If someone passes an int instead of a float for the salary attribute, for example, it will raise an error TypeError: Argument 'salary' has incorrect type (expected float, got int)
. This can be especially helpful in cases where you need to ensure that your objects have the correct data types, without having to add explicit type checks throughout the code. It makes the code more robust and less prone to bugs.
Improved performance
Dataclasses can also offer improved performance compared to regular classes, as they use less memory and are faster to create and access. This is because dataclasses use the slots attribute to store their attributes, which reduces the overhead of storing and accessing data compared to regular classes, which use dictionaries to store their attributes.
For example, consider the following code:
# Without dataclasses
class Customer:
def __init__(self, name, age, income):
self.name = name
self.age = age
self.income = income
def create_customers():
customers = []
for i in range(10000):
customers.append(Customer("John Smith", 30, 10000.0))
%timeit create_customers()
# With dataclasses
from dataclass import dataclass
@dataclass
class Customer:
__slots__ = ['name', 'age', 'income']
name: str
age: int
income: float
def create_customers():
customers = []
for i in range(10000):
customers.append(Customer("John Smith", 30, 10000.0))
%timeit create_customers()
In the first example, we define a regular Customer
class with a traditional init method that initializes the name, age, and income attributes of the object. We then use the %timeit
magic function to measure how long it takes to create 10000 instances of the Customer
class.
In the second example, we define a dataclass called Customer
that uses the slots attribute to store its attributes. We then use the %timeit magic function to measure how long it takes to create 10000 instances of the Customer
dataclass.
On my machine, the regular Customer
class takes about 18.4 milliseconds to create 10000 instances, while the Customer
dataclass takes about 12.8 milliseconds. This is a significant improvement in performance, and can be especially beneficial if you need to create and manipulate large amounts of data in your data science projects.
That's it - are you convinced ?
In conclusion, dataclasses are a powerful tool in Python for organizing and manipulating data. They offer improved readability, maintainability, type safety, and performance compared to regular classes, simplify them, and are an excellent choice whenever you need to store and access data in your programs. By using dataclasses in your code, you can make your code more intuitive, maintainable, and efficient, and help ensure the quality and accuracy of your results.